Getting started with your first .NET project in 2024 (Part 2)
A brief tutorial for someone looking to get started with first .NET project in 2024 (Part 2)
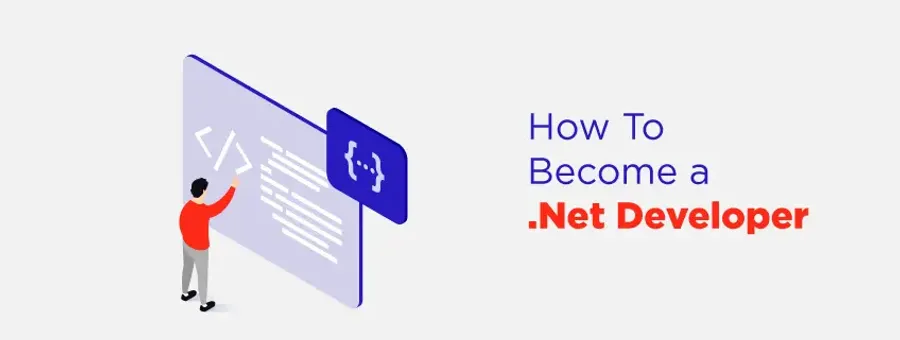
This blog post is continuation of the blog post where we create a new .NET console application project. You can find the link below:
In this tutorial, we will go over the two files that are created when we create a new .NET console application. So, let’s get into it.
Program.cs file
Since release of .NET 6, the Program.cs file has been simplified a lot. The file should simply have the following line of code in it.
Console.WriteLine("Hello, World!");
This line simply prints the text Hello, World!
as a new line in the console when the application is run. If you are familiar with other programming languages, like Python, this is similar to simply calling print("Hello, World!)
. The identifier Console
in this line is a part of System
namespace which is part of the dotnet package. We will discuss why we do not need to specify System.Console.WriteLine
as part of explaining the Demo.csproj
file.
Note: We will discuss namespaces in a future tutorial
Project (.csproj) file
The project (.csproj) files for .NET projects are XML format files with basic information about the project and what is required to build and run the application. Here are the contents of a very basic project file.
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>net8.0</TargetFramework>
<OutputType>Exe</OutputType>
</PropertyGroup>
</Project>
As you can see, the root element of the xml file is a Project element, which is required, with an optional Sdk
attribute that specifies which SDK (and version, if specified) to be used. There are a few different SDKs available to choose from and you can find the official list along with information about each at the link below: Available SDKs
Inside the Project element is a PropertyGroup
element. This element is required as a parent for all the Property
elements for the project.
The next line specifies the TargetFramework
property of the project. This is the target framework that the project will be built against. In order to build this project, you require this version of dotnet SDK installed and to be able to run the built project, you need the same version runtimes installed on the target machines. In our example, we are targeting net8.0
version, which is the latest release at the time of writing.
OutputType
property specified what type of output files will be generated to run the project, once the project is built and bundled. In our example, we are using an executable
here to create as we want to be able to execute the built project.
This is all that is needed to create a basic .NET project. But as the project grows, there will be additional items added to the csproj file.
NOTE: Once again, I have ignored the obj
and bin
directories for the time being to keep things simple, as they are a bit more advanced topics. Just note that these are auto generated folders and will automatically update as you work on the project. Except from very rare cases, you will not need to touch these folders.
In the next tutorial, we will add more functionality to this project to resemble a little bit more .